When working with React.js, developers often face a variety of challenges, from managing state efficiently to optimizing performance. In this article, we’ll dive into three common issues in React development and explore practical solutions for each. Whether you’re struggling with prop drilling, handling asynchronous data fetching, or optimizing performance, these techniques will help you write cleaner, more efficient code.
Handling State and Prop Drilling in React
A major challenge in React is managing state across multiple components, especially in large-scale applications. When you need to pass data down several layers of components, you might run into a problem known as prop drilling. This happens when props are passed through many nested components just to reach a deeply nested child, making your code more complex and harder to maintain.
Solution: Lifting State and Using the Context API
A common fix for prop drilling is to lift state up to the closest common ancestor of the components. However, when your component tree is deeply nested, React’s Context API offers a more scalable solution. It allows you to share data across multiple components without passing props down manually.
Here’s an example:
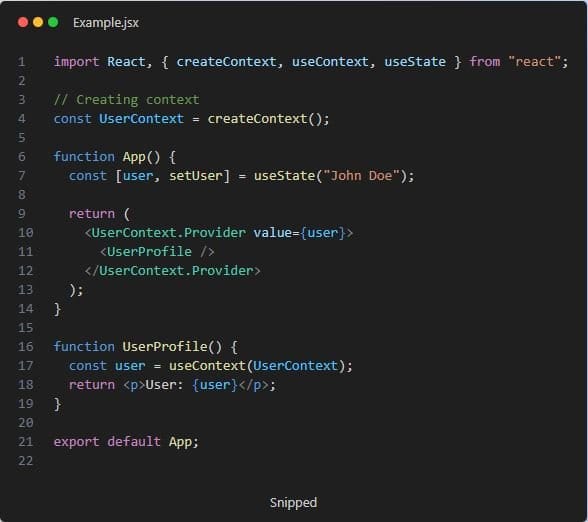
In this example, the Context API allows us to avoid passing props through every component. Instead, UserProfile can directly access the user value from UserContext, making the code cleaner and more manageable.
Dealing with Asynchronous Data Fetching in React
Handling asynchronous data fetching in React can be tricky, especially when working with external APIs. If not handled correctly, it can cause components to render before the data is available, leading to performance issues or errors.
Solution: Using the useEffect Hook for Data Fetching
React provides the useEffect hook to manage side effects like fetching data from APIs. It runs after the initial render and allows you to update the component state once the data is ready.
Here’s a simple example:
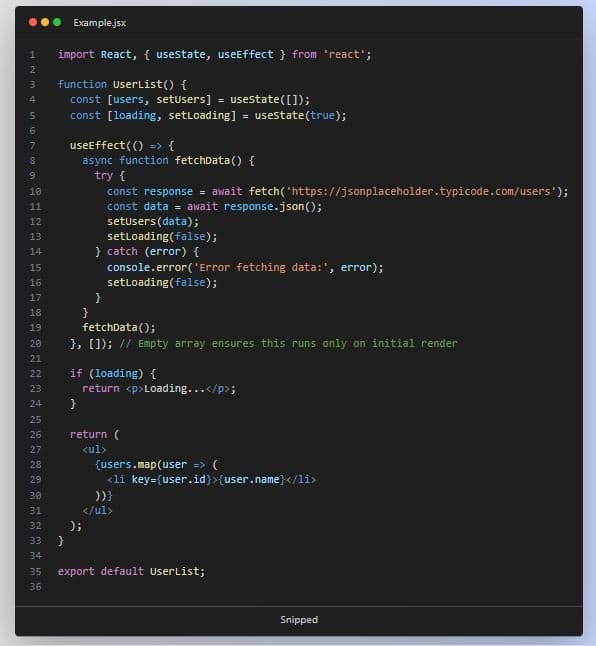
In this example, we use useEffect to fetch the data after the component mounts. We also handle the loading state to prevent the UI from breaking while the data is being fetched.
Optimizing React Performance
As your React application grows, performance optimization becomes essential. If your components re-render unnecessarily, it can slow down your app, especially when dealing with expensive calculations or large datasets.
Solution: Memoization with React.memo and useMemo
Memoization is a great way to optimize performance by caching the result of an expensive operation and returning the cached result if the inputs haven’t changed. React provides two key tools for memoization: React.memo and useMemo.
- Using React.memo to prevent unnecessary re-renders of functional components:
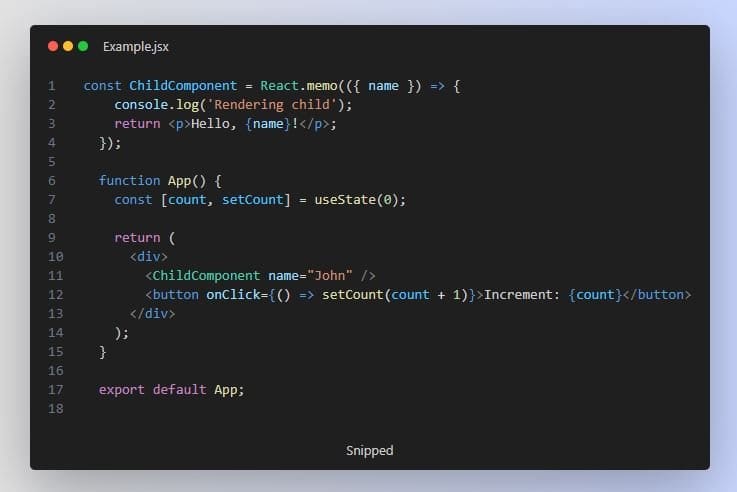
In this example, ChildComponent won’t re-render unless its name prop changes. This helps reduce unnecessary re-renders, which can slow down the app.
- Using useMemo to cache expensive calculations:
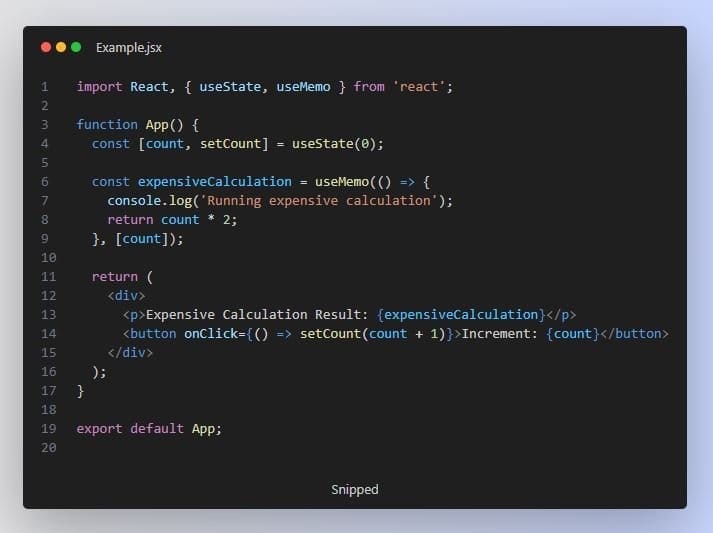
Here, the expensive calculation only runs when count changes, thanks to useMemo. This prevents unnecessary recalculations and optimizes performance.
Conclusion
React.js makes building modern web applications easier, but it still comes with its share of challenges. By understanding how to manage state without prop drilling, handle asynchronous data fetching properly, and optimize performance using memoization, you can write cleaner, more efficient React code. These solutions will help you avoid common pitfalls, improve user experience, and ensure your app remains performant as it scales.
Whether you’re a beginner or an experienced React developer, mastering these problem-solving techniques will make you a more effective developer and help you create high-quality, scalable applications.